Javascript Blackjack
I had no idea what blackjack is (I knew it was some kind of card game), but now, thanks to the step by step from ground zero instructions given by Codecademy, I have a functional Blackjack architecture that works just as intended.
- Blackjack Javascript Code
- Javascript Blackjack Shuffle
- Javascript Blackjack Source Code
- Javascript Blackjack Game
- Javascript Blackjack Code
Free Blackjack Games 2020 - Play blackjack FREE with our instant, no registration games. Enjoy 60+ of the best blackjack games (choose from many variants). Blackjack Game using HTML5, CSS and JQuery. Here's a fun and simple blackjack game I built using HTML5, CSS and JQuery. I'd like to add more features to it like betting, splitting and doubling down and it's on github so anyone can add to it. Follow the link below to play! I'm trying to create a blackjack program for school and I don't understand why my program keeps starting over after i ask for another card once I get my first two cards. Any help would be great. JavaScript closure inside loops – simple practical example. How do I break out of nested loops in Java? Learn JavaScript and Javascript arrays to build interactive websites and pages that adapt to every device. Add dynamic behavior, store information, and handle requests and responses. This course can help marketers and designers upgrade their career and is a starting point for front-end engineers.
I am still not well verse with Blackjack, but I now know the basics of it and can talk about it with people rather than being purely unknown to it.
And guess what, What better way to learn about Blackjack then building it ourselves using a programming language!
Here’s the code that I wrote through Codecademy’s instructions (that was like pseudo code for me as I was completely unknown to Blackjack game)
Expect me soon with more adventures on Codecademy.. Till then adios amigos!
Looking for a new project in JavaScript that I have not done in Python, I got to thinking about arrays.
As JavaScript allows us to generate random numbers and store variables (see “Scissor, Paper, Stone”) it should allow me to also recreate the card game of Blackjack or 21. For more information on Blackjack please see: https://en.wikipedia.org/wiki/Blackjack
The smallest game of Blackjack requires 2 people – a player and a dealer.
Blackjack Javascript Code
The objective of Blackjack is for the player to either score 21 with 2 cards, or get a higher score than the dealer without going over 21. If the player goes over 21 then they lose. If the dealer goes over 21 then the banker loses.
The dealer is going to be controlled by the computer.
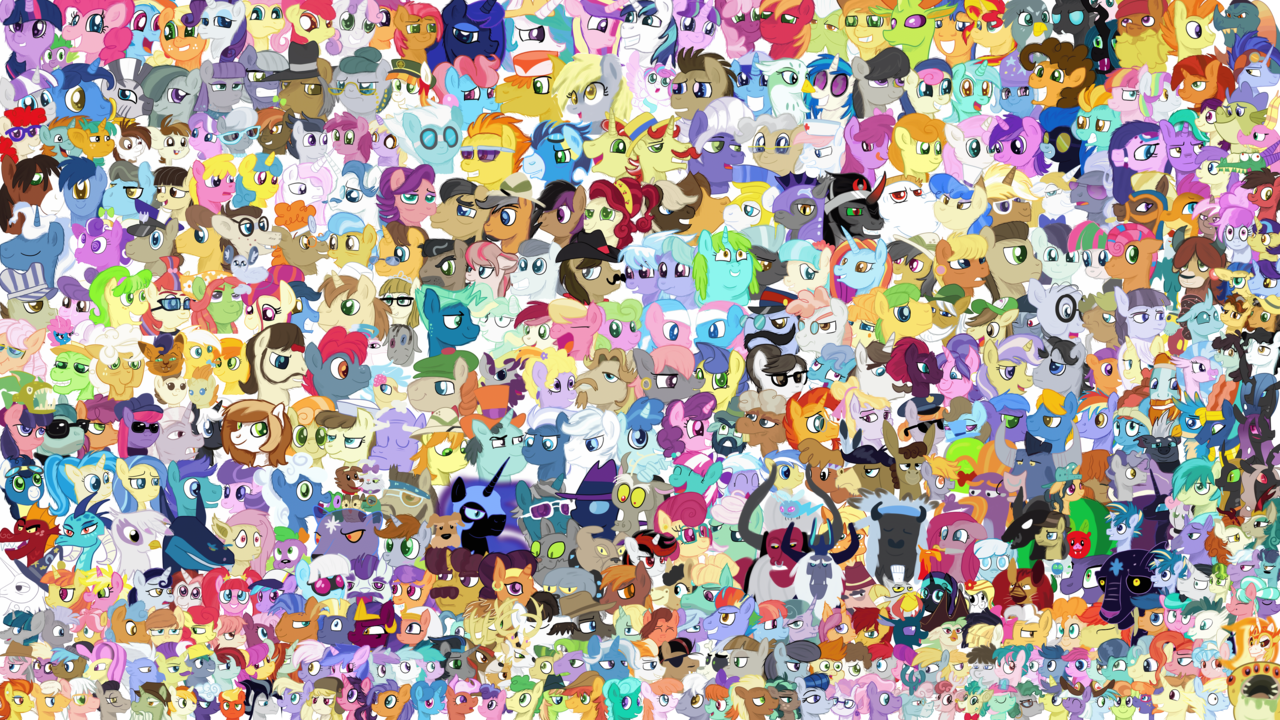
I’m going to use one deck of cards (52 cards). The 52 cards are split into 4 suites (Hearts, Diamonds, Spades, Clubs) with each suite containing 13 cards:

Ace, 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen, King
For this version of Blackjack I’m going to define an Ace as having the value of 1, and the Jack, Queen, King as having the value of 11.
So each suite now has;
Javascript Blackjack Shuffle
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 11, 11, 11
The values can be stored in an array, which we can call “deck”:
// variable to hold the deck of 52 cards
var deck = [1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 6, 6, 6, 6, 7, 7, 7, 7, 8, 8, 8, 8, 9, 9, 9, 9, 10, 10, 10, 10, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11];
We then need JavaScript to randomly choose a card and remove the card from the possible cards that can be played.
Javascript Blackjack Source Code
// geektechstuff
// BlackJack
// variable to hold the deck of 52 cards
var deck = [1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 6, 6, 6, 6, 7, 7, 7, 7, 8, 8, 8, 8, 9, 9, 9, 9, 10, 10, 10, 10, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11, 11];
// variable to choose a random card from the deck
var card = deck[Math.floor(Math.random()*deck.length)],
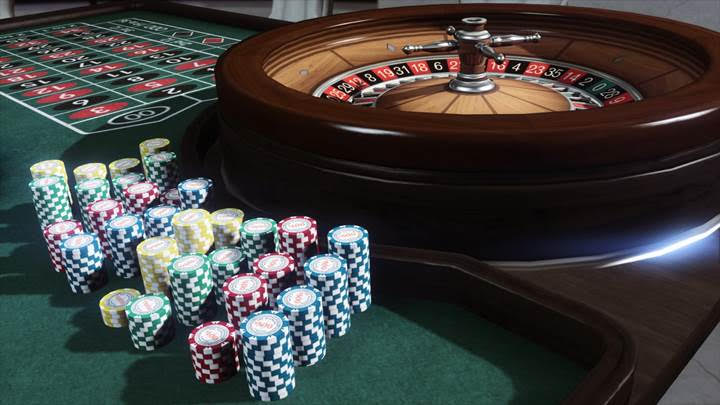
card_remove = card,
Javascript Blackjack Game
position = deck.indexOf(card);
if (~position) deck.splice(position, 1);
Javascript Blackjack Code
// checking that a card is picked and that it is removed from deck
console.warn(card)
console.warn(deck)
Next up I will need to look at storing the card values in the players hand or the dealers hand and checking if the values have gone over 21.